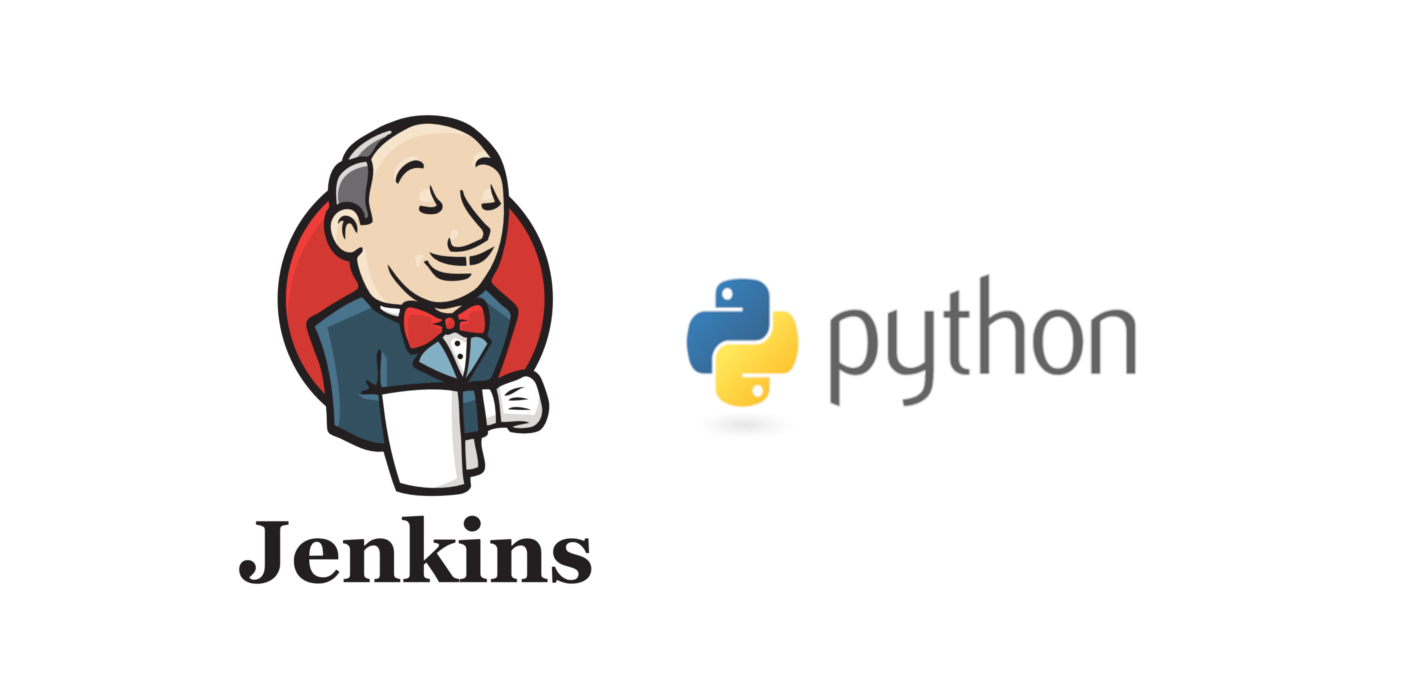
Our Jenkins Pipeline training course is just updated on 2020! – PRESS HERE
Introduction
In the world of Test Automation there are many frameworks available to help testers build their automation infrastructures based on Behavior-driven development (or BDD). We can use Selenium and Cucumber in Java, commit the code in a remote repository and let Jenkins build and run our test project. “Behave” is the framework used in Python language that helps us write test cases in Given-When-Then format and implement the test steps.
When the Python project is run locally, we will end up with a report on which test cases have passed and which test cases have failed. But Python does not use a packaging manager like Maven or a Project Object Model to automatically search for dependencies and download them before the building process starts. Instead, all the packages needed for our Python program to run, have to be installed on the host machine. So, how can we use a Behave project in Jenkins and how can we incorporate it in a Build pipeline?
Server prerequisites
We assume that we already have a simple Python project implemented with the Behave framework, and a Jenkins instance on a server with Ubuntu 18.04. First, we need to make sure that the server can successfully run the project and show us the results of the tests. By updating and upgrading the system on the server, Python v3.6 will be automatically installed. Some development tools that will ensure a robust set-up for our programming environment are build-essential, libssl-dev, libffi-dev and python3-dev. To manage software packages in Python, we will also install pip. The extra packages needed for our project are:
- setuptools, for packaging our project
- selenium, to simulate and automate web browser actions
- behave, the actual BDD framework
The Jenkins instance should also be able to run Python code, so the plugin ShiningPanda is necessary. After installing the plugin we can add Python through the global configuration tool by filling in the path to Python 3 executable, which in the most cases is “/usr/bin/python3”. More information about how to configure the ShiningPanda plugin can be found here https://bit.ly/2F2eqdF
Prepare the Python project
Our Python project will be run on a Linux server with the help of ChromeDriver. Since the server does not have a GUI preinstalled, the project needs to be run in headless mode. Furthermore, there are some other options that we have to add to our driver.py file in the project that instantiates the WebDriver object, to ensure compatibility with the server:
options = Options() options.add_argument('--headless') options.add_argument('--no-sandbox') options.add_argument('--start-maximized') options.add_argument('--disable-extensions') options.add_argument('--disable-dev-shm-usage') driver = webdriver.Chrome("/usr/local/bin/chromedriver", chrome_options=options)
In order to test if the server can run our project with the current configuration, we can upload all project files to a temporary directory and run the following command:
behave -i test.feature --junit
If we see the the logs of the test steps and the results of the test cases on the screen, we are ready to continue.
Configure the Jenkins Freestyle job
There are two kinds of jobs that we can configure in Jenkins. Let’s start with creating a Freestyle job. We need to follow these basic steps in Jenkins:
- Add the repository URL that contains the Python project, to the Source code management section
- Add a Build step, select Virtualenv builder and enter the following commands: pip install behave, pip install selenium
- Add a build step, select Python builder and enter the command: behave -i test.feature –junit
This will enable the ShiningPanda plugin to create a virtual environment with the appropriate Python packages and then run the project with the same command we used earlier. Finally, we can:
- Add a post build action: Publish JUnit test result report and fill in the tests report XML directory as it is configured in the Python project
When we Build the Freestyle job, we will see that a Test Result option is available on the Build details page and from there we can see the report on how many test cases have passed and how many test cases have failed.
Working with a Pipeline
If we do not want a Freestyle job and instead we are only working with Pipelines, we can create a simple Pipeline job in Jenkins, select Pipeline script from Git and enter the Git repository URL of our Python project. In the Script path section we should enter the name of a new file that we will create for configuring the pipeline. We will use the the Jenkinsfile.groovy name, so in our repository we will need to create this file with the following content:
node() { stage('checkout') { deleteDir() checkout scm } stage('build') { sh "behave -i test.feature --junit" } stage('publish') { junit 'reports/*.xml' } }
Since the server is already configured with all the required Python packages, the shell command will execute successfully and the Python project will produce the same results as before, visible on the Test Result option on the Build details page.
Conclusion
In this article we examined the case when testers use Python and the Behave framework to build their test automation infrastructure and implement the BDD test cases and test steps. Then, we showed how to configure the Jenkins server with the required packages and implemented a Freestyle job and a Pipeline job in Jenkins, to Build our project and see the test results. This is a good example to show that Jenkins can support different languages and technologies with just a few configuration changes.